Create a Blockchain with Python
No matter what your area of expertise sooner or later you will run across the concept of Blockchain. Be it IT, Development, QA, DevOps or SRE, Blockchains are making their way into all facets of technology. There are multiple public blockchains available like Ethereum, Binance, Bitcoin, Polygon, Solana, WAX, EOS. Or, depending on then need, you can also roll out your own private chain.
All of these blockchains will have features and quirks of their own like Proof of work, Proof of Stake etc. However at core all blockchains function in similar fashion. They write data or “transactions” into blocks.
So let’s try and understand blockchains by building our own using Python !
Note: I do not explain how to setup a Python virtual env to run this example. The only dependency this script has is loguru, which is used to print pretty log statements. If you want to avoid installing it, just replace all the “log.info()” with “print()”.
At the heart of an blockchain is the block. A block, in blockchain, accepts a series of transactions AND the hash of the previous block in the chain, combines them both and writes them inside of itself. Why the hash of the previous block ? We will discuss that in a bit. First let’s write out block class.
As you can see see our block takes the hash of the previous block and an array of transactions as an argument. Our block is doing two things. It is converting the transactions to strings and appending the previous hash to the transitions and storing it as data and it is also storing the hash of the whole data as the block hash. That’s all there is to the block !
Now let’s create a chain class that will make use of the block class to actually create a chain. Now this class will do some heavy lifting. So the code is lengthier.
As you can see when you initialize the chain, it maintains the copy of the chain in an array called chain. The chain also keeps count of all the transactions written to it. We are also making the transactions per block parameter tunable. Currently it is set to 10. This means after every 10 transactions the chain will product a new block. Feel free to experiment with this parameter. And lastly we have a buffer which is an array where transaction are stored before being written to the block.
Now is a good time to discuss blocks, hashes and what makes blockchains so special. As we saw in our Block class, a block combines the hash of the previous block along with its own data to come up with its own hash. This hash will then be forwarded to the next block to form a chain. If somehow the data in one block is changed, then the hash of the block will change and it won’t match the hash stored in the next block and it will break the whole chain ! That’s why blockchains are secure and transactions once written to blockchain are permanent. But what about the very first block in the blockchain ? It has no previous block and hence no hash for it.
The first block in a blockchain is special and is called a genesis block. This is because it has no preceding block and hence no hash value can be passed to it. Usually no transactions are written to it. In our case we are manually generating it with a hash value of “Genesis Block” and a single transaction of “[Genesis Block]”.
Besides creating genesis block our chain class has a few other useful functions namely one to get the last_block, one to write_transaction and one to print_chain. The write_transaction will generate a new block every time transactions_per_block are written to a block.
And lastly we create a main.py to create a chain and then write some transactions to the chain.
The transactions are simply a for loop that counts till 100 and calls the write_transaction on the chain. If you run main.py, you will see that a new block is generated every time transaction_per_block is greater than 10 (or whatever number you set it to).
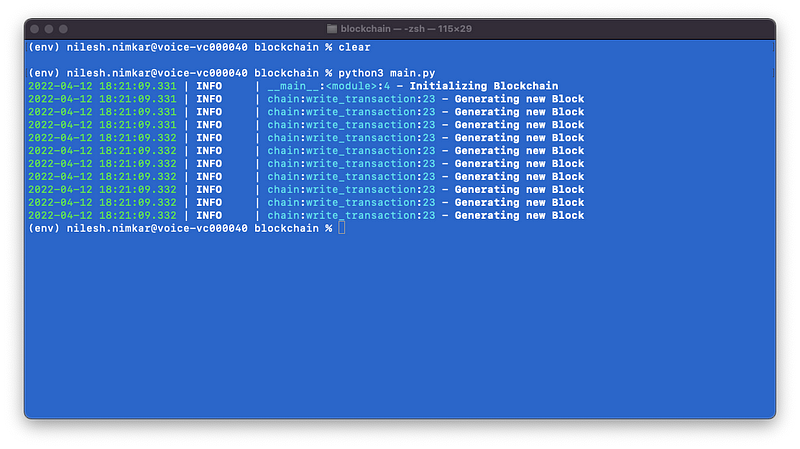
And last but not the least you can uncomment the print_ chain to examine the chain data !!
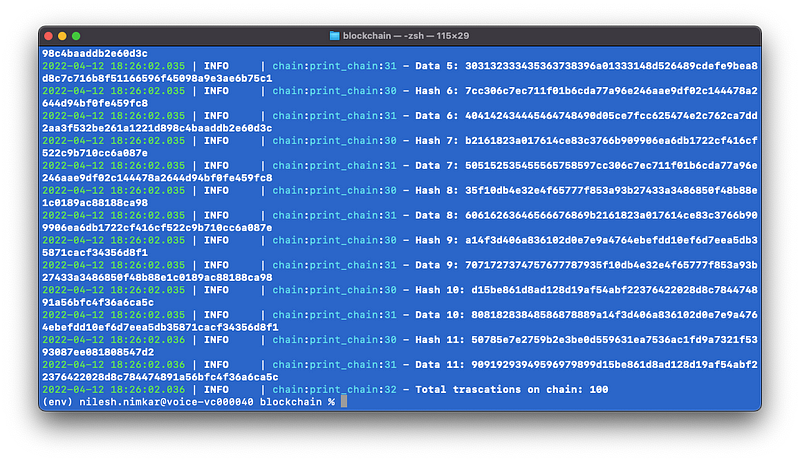
The complete code can be found here https://github.com/nimkar/blockchain
If you liked this article, consider signing up for new content below.
Comments ()